Alma Developers visited biggest React conference in the Europe React Amsterdam on 21.4.2017. Before we dive into the blog post about the conference, here are some photos from our trip to Amsterdam:
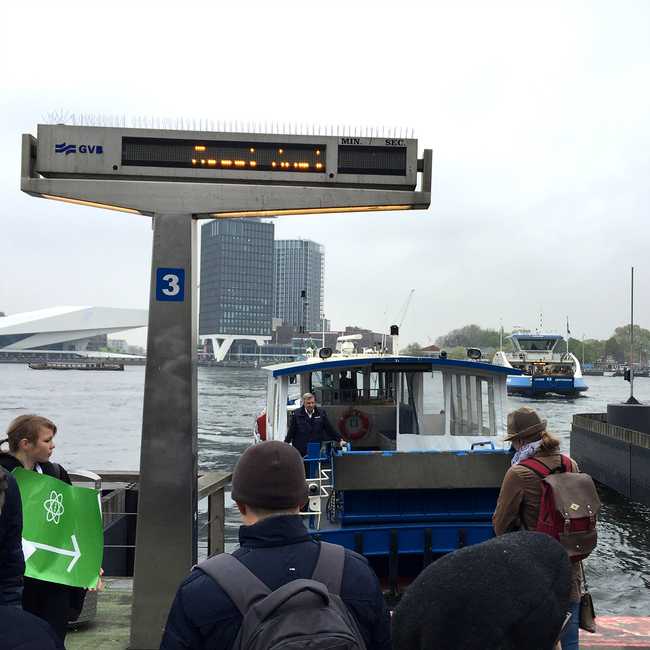
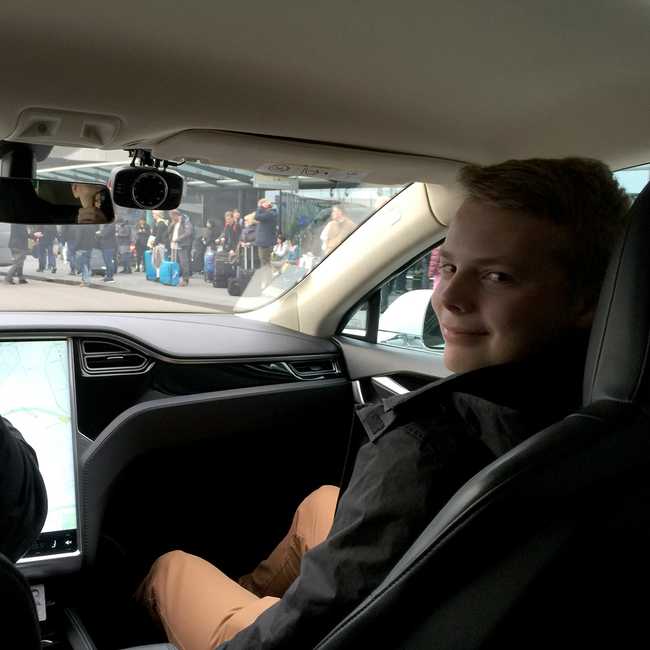
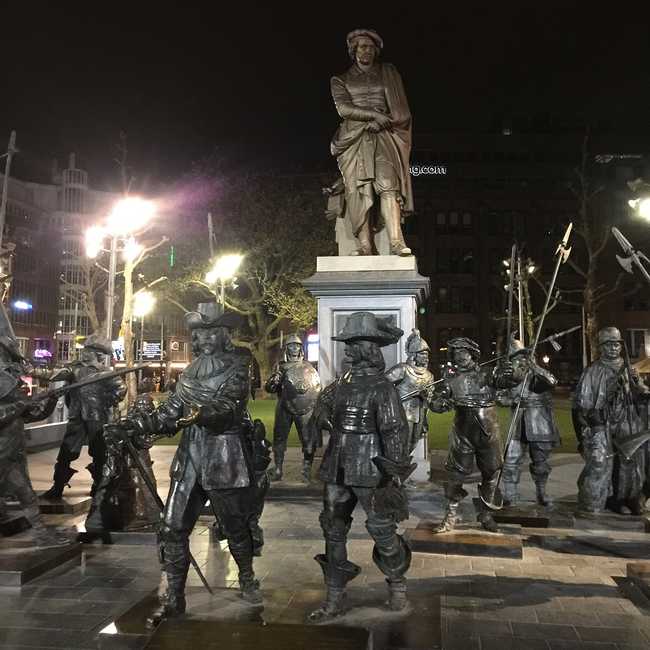
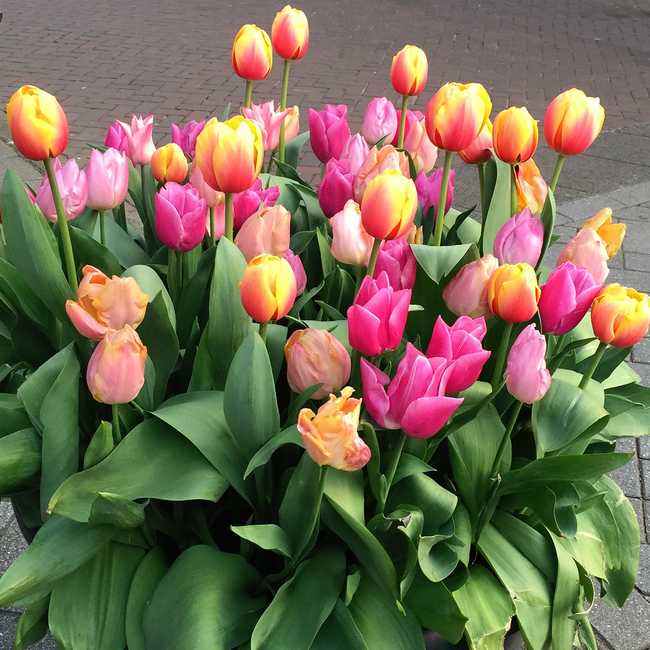
Conference Day
First I was going to cover all presentations, but not all them were that great or interesting to us; There where some nice talks about Virtual Reality, custom renderers with Fiber, Flow, “from X to React” and whole React Native track. So if you are into those check Youtube for React main or native tracks. Instead I will focus this blog post only on those presentations which we found most interesting.
Styled Components
First presentation was about styled-components by Max Stoiber. We actually missed the beginning of the presentation, because it started ahead schedule (or maybe we were just late 😀).
With styled components you can actually write CSS inside JS and you have power of components. Styled components remove the need for using class names in components. If you use class mapping to style only once you really don’t need them. Instead you define styles within a component by following method:
import styled from 'styled-components';
const Title = styled.h1`
font-size: 1.5em;
text-align: center;
color: palevioletred;
`;
const Wrapper = styled.section`
color: palevioletred;
padding: 4em;
width: 100%;
height: 100%;
background: papaywhip;
`;
const App = () => (
<Wrapper>
<Title>
Hello World from styled component
</Title>
</Wrapper>
);
ReactDOM.render(
<App />,
document.getElementById('#app');
);
There’s no magic in this syntax; Prefixing template literals (denoted by back-ticks ``
) with a function name - i.e. “tagging” - is actually an ES6 feature called tagged template literals.
If you’re still doing styles in scss or css files, you’re totally missing out! There is no need for style preprocessors any more: You can do many things with just JavaScript and for most demanding tasks (think of scss mixins & helper functions) you can just import library like polished. For themes, just import ThemeProvider
from Styled Components which allows different styling for same component. We definitely will try this on our new React stack, where we will try to have shared components between different sites/brands.
Next version (coming in next 2 weeks) will include support for server side rendering. That will export only those styles that you use! Don’t hold your breath while waiting. Styled components seem to be ready for prime time, especially when next release will have support for server side rendering (SSR). SSR is great feature and you can bet great performance benefits, but nothing good comes without cost. Especially for sites where ads are great part of financing it gets tricky pretty fast. Still it was great to hear experiences from others on this field.
If you want to know more there’s nice article how to style React components with styled-components or just visit official site.
Lightning talk: Advanced SSR Caching with React
In his lightning talk Advanced SSR Caching with React Robert Haritonov told how Zeever has chosen React over other JS libraries mainly for server-side rendering capabilities. He said that server-side rendering is supported by React, but it’s not first class citizen and there’s not that many community developers focusing on making it faster. Most efficient approach on caching is do it on component level. One of the biggest players on SSR at scale is Wallmart and they have own Electrode platform. With SSR and caching container component you can squeeze response time from 200ms down to 15ms \o/
SSRCaching.cacheConfig = {
components: {
"Accommodation": {
strategy: "simple",
enable: true,
genCacheKey: (props) => props.accommodationId
}
}
};
<Route
path="/accommodation/:juice/:accommodationId"
component="Accommodation"
onEnter="{onEnter(toAccommodation)}"
>
....
</Route>
Caching is done then on route level. There has been some difficulties with this solution and recently they have looked if Formidable/rapscallion could solve some of these. Rapscallion is asynchronous which allow you cache on remote servers and to have common cache for cluster with streaming support.
render(<MyComponent {...props} />)
.toPromise()
.then(htmlString => console.log(htmlString));
<Accommodation cacheKey={ `Route:${heliosId}` }>
....
</Accommodation>
Zoover is on beta stage and have started to experiment SSR with real users.
Make Linting Great Again
Lightning talk:There’s great project to manage git hooks on your project called Husky
{
"scripts": {
"precommit": "eslint ."
}
}
For large projects it will take some time to run linting on all files. That’s why Andrey Okonetchnikov created Lint-staged project to lint only staged files. And you can then add auto fixing for linting errors and reformat your code with GREAT project called prettier. For my oppinion Prettier with standard is great tool for auto formatting and fixing your code. Like gofmt for Golang it just makes everyone’s code readable and you can skip all tabs vs spaces fights for good. Both tools are opinionated so you might not agree on all things but in the end it matters the most that all code is formatted at the same way. So much less mental processing for decypting different formatting styles.
{
"scripts": {
"precommit": "list-staged"
},
"lint-staged": {
"*.js": [
"prettier --write",
"git add"
]
}
}
Test it like it’s 2017
Testing is really hard. It’s time consuming. Test environments are complex to setup. You get false positives. Still testing is important! You catch bugs before production. Tests gives confidence to accept pull requests. Tests can be your documentation. Despite the unit tests are losing their appeal. 2009 unit tests were popular because they were fast to run.
Michele Bertoli from Facebook believes that one tool to solve all problems is JEST, which he goes through in his presentation Test it like it’s 2017. It has been build developer experience in mind. It tries to make testing painless. Jest promises zero configuration, fast because tests run in parallel. Still it doesn’t leak information because tests are sandboxed. It gives you instant feedback if you run Jest in watch mode! It will run failed tests first. It has built-in code coverage reports, mocking library and it works with typescript.
One single reason it those were not appealing enough is SNAPSHOT testing. At the first run it will take “picture” = complete html code from that component. On next run it will take new picture and then compare these two outputs and tells what has changed (diff). It just tells that component has changed.
test('test-renderer', () => {
const tree = render.create(
<Button disabled primary text="Click me!" />,
).toJSON()
expect(tree).toMatchSnapshot()
})
If you change class name your test will fail. Jest will then give you option to update snapshot if this was change that you wanted to happen. For this reason you HAVE TO COMMIT YOUR SNAPSHOTS! You can test even Redux actions with Jest. It even works with styled-components with jest-styled-components. So you can extend Jest to test any serializable value.
What’s next?
“The best testing strategy is not writing tests” - Michael Bartoli Dec 2016
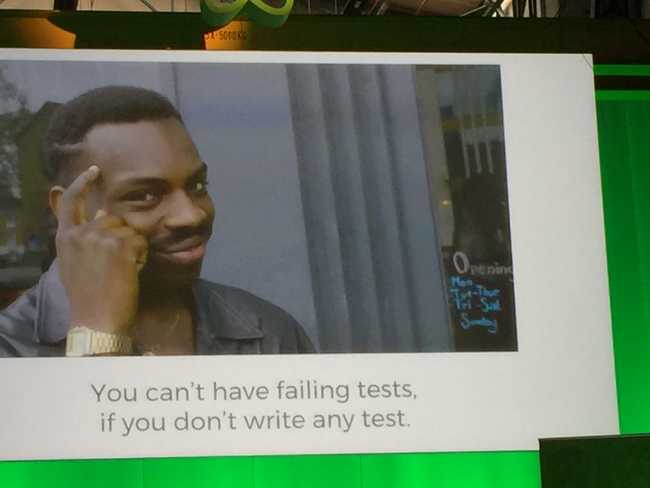
This doesn’t mean that you don’t have tests, you just don’t write tests.
Startup phase is style guide. React styleguidist will generate styleguide out of your components. Green line on every component on styleguidist will tell you that Jest snapshot will match to current state of component! You can edit test case and update snapshot from styleguidist UI. React storybook has similar functionality with storyshots.
Another great tool for React Fix It. It will help you reproduce issues (bugs) that your application. If you have it listening on app it will write test snippet of failing functionality. This is pretty AWESOME \o/
Recap:
- Find a painless way to write tests
- Write more tests
- Have fun!
MobX
MobX like RxJS/observables is great tool but similar to Redux it’s not THE hammer for all different nails. Observables adds extra cognitive switch. If you’re into Mobx you should watch Michel Weststrate’s presentation.
Summary
React family has grown nicely and thanks to it’s very open community it will be around at least for some time. Core React team has said that Facebook has over 30 000 React component so there will be always upgrade path. That’s sadly not the case for all 3rd party libraries, but for sure you can count that React Fiber and what comes after that will not brake your components. Or if it will brake you get codemod to fix them.
Overall React Amsterdam treated us great. So we definitely ask from our developers again next year who want to visit lovely Amsterdam.